s01: Python Basics
Contents
s01: Python Basics#
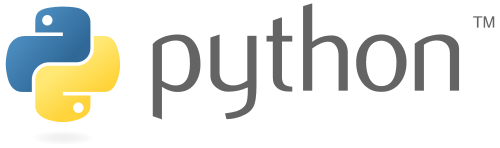
Objectives#
Get a very short introduction to Python types and syntax
Be able to follow the rest of the examples in the course, even if you don’t understand everything perfectly.
We expect everyone to be able to know the following basic material to follow the course (though it is not everything you need to know about Python).
Scalars#
Scalar types, that is, single elements of various types:
i = 42 # integer
i = 2**77 # Integers have arbitrary precision
g = 3.14 # floating point number
c = 2 - 3j # Complex number
b = True # boolean
s = "Hello!" # String (Unicode)
q = b'Hello' # bytes (8-bit values)
Collections#
Collections are data structures capable of storing multiple values.
l = [1, 2, 3] # list
l[1] # lists are indexed by int
print(l)
l[1] = True # list elements can be any type and changes now
print(l)
print('\n')
d = {"Janne": 123, "Richard": 456} # dictionary
d["Janne"]
print(d.keys()) # this gets all the keys from a dict
print(d.values()) # this extract all values from dict
print('\n')
[1, 2, 3]
[1, True, 3]
dict_keys(['Janne', 'Richard'])
dict_values([123, 456])
Control structures#
Python has the usual control structures, that is conditional statements and loops. For example, the if
statement:
x = 2
if x == 3:
print('x is 3')
elif x == 2:
print('x is 2')
else:
print('x is something else')
x is 2
While while
loops loop until some condition is met:
x = 0
while x < 42:
print('x is ', x)
x += 5
x is 0
x is 5
x is 10
x is 15
x is 20
x is 25
x is 30
x is 35
x is 40
For for
loops loop over some collection of values:
xs = [1, 2, 3, 4]
for x in xs:
print(x)
1
2
3
4
You can nicely iterate over elements of a list using the for
loop independet of the datatype it is holding:
l = [1,'b',True,]
for e in l:
print(e)
1
b
True
A common need is to iterate over a collection, but at the same time also have an index number. For this there is the enumerate
function:
xs = [1, 'hello', 'world']
for ii, x in enumerate(xs):
print(ii, x)
0 1
1 hello
2 world
List comprehensions#
List Comprehensions are an alternative way to elegantly create lists. List Comprehensions are basically loops, but you can make them syntactically more compact (strictly speaking, List Comprehensions are not really necessary, because you can’t do anything with them that wouldn’t be possible otherwise).
Let’s take as an example a list of square numbers from 0 to 9. With a normal loop you would create this list like this
squares = [] # wir beginnen mit leerer Liste
for x in range(10):
squares.append(x**2) # Liste wird befüllt
squares
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
The same result can be written much shorter with a List Comprehension:
squares = [x**2 for x in range(10)]
squares
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
The ingredients of a List Comprehension are:
Two enclosing square brackets (which, after all, define a list).
An expression
A for statement (which iterates over an object)
Optionally an if-condition
Optionally further for-statements
A relatively complex example might look like the following:
[(x - 1, y - 2) for x in [1, 2, 3] for y in [3, 1, 4] if x != y]
[(0, 1), (0, 2), (1, 1), (1, -1), (1, 2), (2, -1), (2, 2)]
Functions and classes#
Python functions are defined by the def
keyword. They take a number of arguments, and return a number of return values.
def say_hello(name):
"""Say hello to the person given by the argument"""
print('Hello', name)
return 'Hello ' + name
str_out = say_hello("Anne")
Hello Anne
Classes are defined by the class
keyword:
class Hello:
def __init__(self, name):
self._name = name
def say(self):
print('Hello', self._name)
h = Hello("Richard")
h.say()
Hello Richard