s01: Jupyter Notebooks
Contents
s01: Jupyter Notebooks#
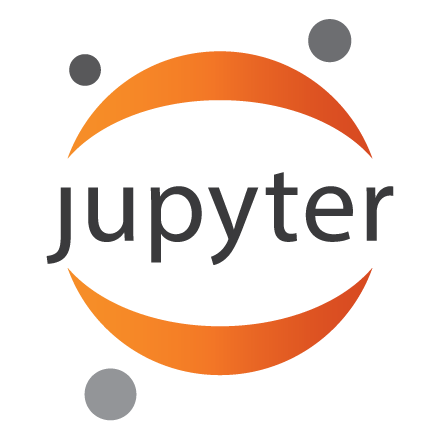
This is a quick introduction to Jupyter notebooks.
Objectives of this session:#
Be able to work with jupyter notebook interface
Know how to create and run cells
Understand difference between markdown and code
Learn how to use the documentation and shortcuts
Cells#
Cells, can be markdown (text), like this one or code cells.
Markdown cells#
For communicating information about our notes, markdown cells are helpful. They apply basic text formatting such as bold, italics, headings, links, and photos. To view the plain text in any of the cells in this section, double-click on any one of them. Run the cell to observe how the Markdown formatting appears.
This is a heading#
This is a smaller heading#
This is a really small heading#
We can italicize my text either like this or like this.
We can embolden my text either like this or like this.
Here is an unordered list of items:
This is an item
This is an item
This is an item
Here is an ordered list of items:
This is my first item
This is my second item
This is my third item
We can have a list of lists by using identation:
This is an item
This is an item
This is an item
This is an item
This is an item
We can also combine ordered and unordered lists:
This is my first item
This is my second item
This is an item
This is an item
This is my third item
We can make a link to this useful markdown cheatsheet as such.
Code Cells#
Code cells are cells that contain python code, that can be executed. Comments can also be written in code cells, indicated by ‘#’.
# In a code cell, comments can be typed
a = 1
b = 2
# Cells can also have output, that gets printed out below the cell.
print(a + b)
3
# Define a variable in code
my_string = 'hello world'
# Print out a variable
print(my_string)
hello world
# Operations that return objects get printed out as output
my_string.upper()
'HELLO WORLD'
# Define a list variable
my_list = ['a','b','c']
# Print out our list variable
print(my_list)
['a', 'b', 'c']
Accessing Documentation#
# Get information about a variable you've created
my_string?
Names#
Names in Python are nothing more than names for certain objects (in other programming languages, names are usually called variables). For example, the assignment a = 1
can be interpreted as giving the name a
to object 1
.
Names can contain letters and digits, but they must not start with a digit. In principle, lowercase letters should be used (Python is case-sensitive). Names may be of any length, but should be chosen as short as possible (but as long as necessary). In addition, the character _ (the underscore) may be used to make a name more readable, e.g. to separate parts of words.
Names should be chosen sensibly, i.e. they should document the usage or the content (i.e. short names like i, n and x are only sensible in exceptional cases). It is also reasonable to use English names.
Examples for valid names are:
number_of_students_in_class = 23 # to long
NumberOfStudents = 24 # Words should be separated with _
n_students = 25 # good name (short, meaningful)
n = 25 # less good (too unspecific), but OK in some cases
Data types#
These are the main data types in python we are going to use
bool (Logical)
numeric
int (Integers)
float (Decimal numbers)
str (String/ Zeichenkette)
list (List of objects)
We can use the command type()
to find out the data type of a variable.
b = True
type(b)
bool
a = 17
type(a)
int
a = 23.221
type(a)
float
Due to the limited computational precision with which computers represent decimal numbers, rounding errors may occur (decimal numbers generally cannot be represented exactly). Example:
0.1 + 0.2 == 0.3
False
0.1 + 0.2
0.30000000000000004
s1 = "Python"
s2 = 'Python'
print(type(s1))
print(type(s2))
<class 'str'>
<class 'str'>
k = [1, 2, 18.33, "Python", 44]
type(k)
list
Autocomplete#
# Move your cursor just after the period, press the first letter of the command you want to execute or tab for all avaliable commands, and a drop menu will appear showing all possible completions
np.
Input In [17]
np.
^
SyntaxError: invalid syntax
# Autocomplete does not have to be at a period. Move to the end of 'ra' and hit tab to see completion options.
ra
# If there is only one option, tab-complete will auto-complete what you are typing
ran
Kernel & Namespace#
You do not need to run cells in order! This is useful for flexibly testing and developing code. The numbers in the square brackets to the left of a cell show which cells have been run, and in what order. However, it can also be easy to lose track of what has already been declared / imported, leading to unexpected behaviour from running cells.
The kernel is what connects the notebook to your computer behind-the-scenes to execute the code. It can be useful to clear and re-launch the kernel. You can do this from the ‘kernel’ drop down menu, at the top, optionally also clearing all ouputs.